[Leetcode] 814. Binary Tree Pruning explained
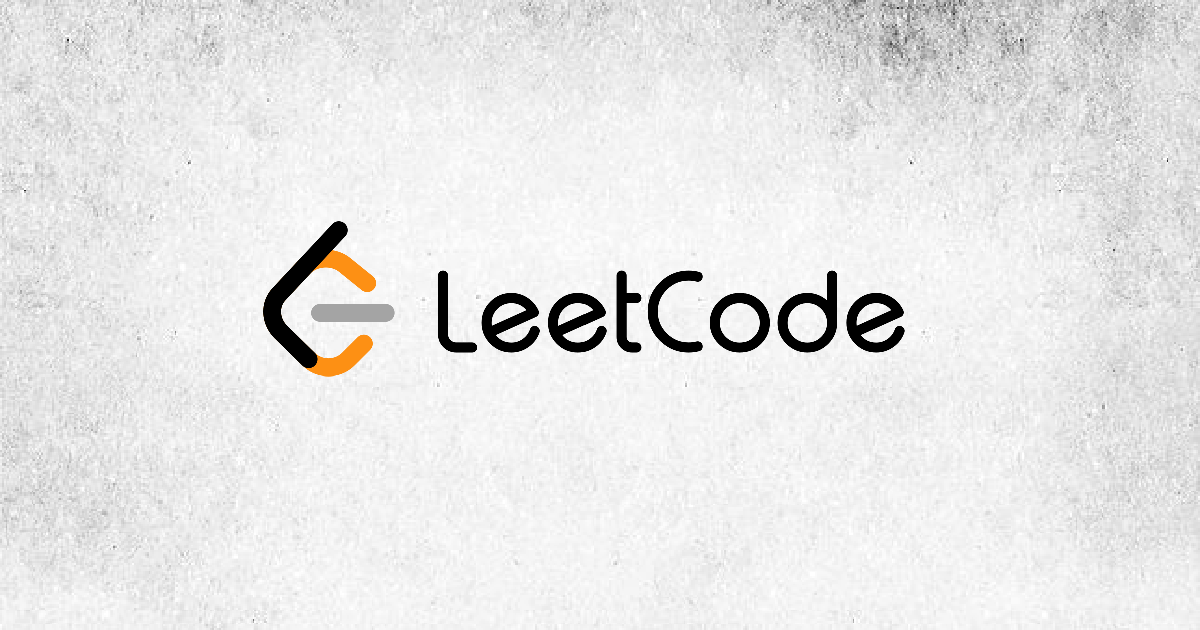
Problem
Approach
For each tree node, repeat below process.
- Prune left child.
- Prune right child.
- If left and right child is
null
and this node doesn’t have value1
, returnnull
(prunning).
Code
/**
* author: jooncco
* written: 2022. 9. 6. Tue. 13:14:14 [UTC+9]
**/
class Solution {
private final int TARGET_VALUE = 1;
public TreeNode pruneTree(TreeNode root) {
if (root == null) return null;
root.left= pruneTree(root.left);
root.right= pruneTree(root.right);
if (root.left == null && root.right == null && root.val != TARGET_VALUE) return null;
return root;
}
}
Complexity
- Time: \(O(n)\)
- Space: \(O(n)\)
Leave a comment